Creating Advanced ASP.NET MVC Controls (Part 2, Finished Debugger)
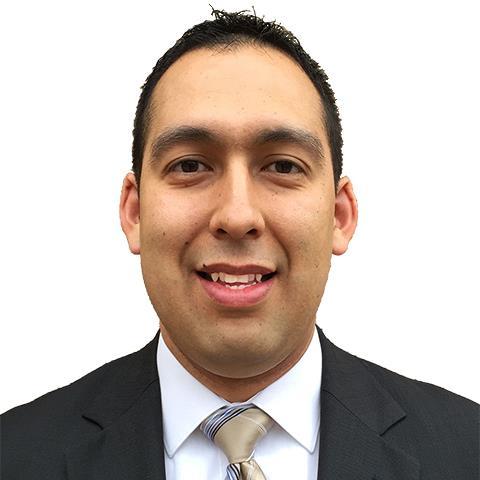
Purpose
As mentioned in the previous post, in order to create good client side controls that interact well with the ASP.NET MVC system, we need to have a way to visualize data that the control either generates, or passes to the controllers. I found this difficult to acheive in IE as well as Firefox. I did not need/want all of the complexities of Firebug or IE's Developer tools (which are great btw). I just wanted to see my data! The problem with the previous version of the debugger was that it was too dang simple! If I were to use it like this:
_('Test').clear()
.write('(Simple)')
.set(complexArray)
.write('(Complex Array)')
.set({ one: { a: 'Hospital', b: 4, c: {x:3,y:5}}, two: 'test'})
.write('(Complex Object)')
.set(function(arg) { alert('HI!' + arg); })
.write('(function)')
.set({ one: new Array('Happy', 'debugger', 'array'), two: 'test'})
.write('(Complex Object)')
.set(
new Array(
new Array(
{a: 1, b: 2, c: 3},
{a: 4, b: 5, c: 6},
{a: 7, b: 8, c: 9}),
new Array(
{a: 'one', b: 'two', c: 'three'},
{a: 'four', b: 'five', c: 'six'},
{a: 'seven', b: 'eight', c: 'nine'})))
.write('(Complex Array)');
});
the debugger would simply not be good enough!
On to version 2!
In order to actually print something useful given the complexity of the potential objects, we need to use some recursion. The bottom level of the recursion would simply be printing out simple data types:
- either a function (yes this is a data type),
- a string, or
- a number
The debugger would then simply recurse over arrays and objects down to the base types.
This turned out to be harder because of a simple mistake I made. What is the difference bewteen these two snippets:
Snippet 1
writeArray: function(li, arg) {
for(var i = 0; i < arg.length; i++) {
$(li).append('<span class="index">[' + i + ']:</span>')
this.dispatcher(li, arg[i], true);
$(li).append('<br/>');
}
}
Snippet 2
writeArray: function(li, arg) {
for(i = 0; i < arg.length; i++) {
$(li).append('<span class="index">[' + i + ']:</span>')
this.dispatcher(li, arg[i], true);
$(li).append('<br/>');
}
}
Nothing you say? Nay dear reader, it turns out that snippet 2 is VERY wrong! During recursive calls, the variable i if not re-declared using the var keyword gets reassigned in each recursive call to itself: this leads to a short-circuiting of the for loop.
Final Product
In this version I removed the jQuery UI references because the draggable got annoying. Here are some screenshots:
and
Final Words
In the next post, we will actually start to build a control. I am thinking some kind of advanced date-picker thing-y (that is a technical term) that allows users to toggle multiple dates and add meta information to said dates. Here is the code.
Your Thoughts?
- Does it make sense?
- Did it help you solve a problem?
- Were you looking for something else?